There are times when creating an app, webpage, or anything of the like where you would like all of a certain type of object to have a similar look, and feel. XAML’s answer to this is default styles.
Default styles are the styles that take effect when you do not specify one. Create a button tag, give it no style, and it will use the out of the box default. You may override this default style so that when there isn’t one specified, it will use your default. You do this by creating a style resource with the targettype specified, but with no key specified.
<Style TargetType="Button"> {...Setter Collection...} </Style>
If this tag was placed in the Application.Resources tag, this style would be the one that takes effect whenever you specify a button without specifying a style. Great…, but you have a problem. This problem comes when you have a panel where you need the buttons to have the similar feel but need just a slight modification. How could you do it? One way to solve the problem is to specify a key attribute on the style tag, rewrite every button to specify that style, and then create a new resource based on that style, and give the buttons you need to have the slightly modified style the key to the second resource created. Does that sound like fun? Doesn’t to me. Sounds like a nightmare. There must be a better way, and there is! When you use the BasedOn attribute of the Style tag,
just reference the type of object instead of the name of a style. Done.
This technique can be used to create a new named style or a new default for all children of a panel. Let’s see an example of this done.
<Border BorderBrush="Black" BorderThickness="1" Height="70" Width="150"> <Grid ShowGridLines="True"> <Grid.Resources> <!-- Here is the new default style for an Ellipse. This will be used by all Ellipses that are children of this Grid. --> <Style TargetType="Ellipse"> <Setter Property="Fill" Value="Red"/> <Setter Property="Height" Value="20"/> <Setter Property="Width" Value="30"/> </Style> <Style TargetType="Grid"> <Setter Property="HorizontalAlignment" Value="Center"/> <Setter Property="VerticalAlignment" Value="Center"/> </Style> <!-- Here is a style based on the default style I specified above slightly modified, and given a key --> <Style TargetType="Ellipse" BasedOn="{StaticResource {x:Type Ellipse}}" x:Key="blueEllipse"> <Setter Property="Fill" Value="Blue"/> </Style> </Grid.Resources> <Grid.ColumnDefinitions> <ColumnDefinition/> <ColumnDefinition/> <ColumnDefinition/> </Grid.ColumnDefinitions> <Grid> <Ellipse/> </Grid> <Grid Grid.Column="1"> <Grid.Resources> <!-- Here is a new default style based on the default style I specified above slightly modified. This will be used by all Ellipses that are children of this Grid. --> <Style TargetType="Ellipse" BasedOn="{StaticResource {x:Type Ellipse}}"> <Setter Property="Fill" Value="Green"/> </Style> </Grid.Resources> <Ellipse/> </Grid> <Grid Grid.Column="2"> <Ellipse Style="{StaticResource blueEllipse}"/> </Grid> </Grid> </Border>
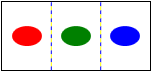